If your website has hundreds of pages with many
visitors everyday, you might want to implement some sort of caching
mechanism for your website to speed up page loading time. Each
client-server request consist of multiple database queries, server
response and the processing time increasing overall page loading time.
The most common solution is to make copies of dynamic pages called cache
files and store them in a separate directory, which can later be served
as static pages instead of re-generating dynamic pages again and again.
Understanding Dynamic pages & Cache Files
Cache files are static copies generated by dynamic pages, these files are generated one time and stored in separate folder until it expires, and when user requests the content, the same static file is served instead of dynamically generated pages, hence bypassing the need of regenerating HTML and requesting results from database over and over again using server-side codes. For example, running several database queries, calculating and processing PHP codes to the HTML output takes certain seconds, increasing overall page loading time with dynamic page, but a cached file consist of just plain HTML codes, you can open it in any text editor or browser, which means it doesn’t require processing time at all.Dynamic page :— The example in the picture below shows how a dynamic page is generated. As its name says, it’s completely dynamic, it talks to database and generates the HTML output according to different variables user provides during the request. For example a user might want to list all the books by a particular author, it can do that by sending queries to database and generating fresh HTML content, but each request requires few seconds to process also the certain server memory is used, which is not a big deal if website receives very few visitors. However, consider hundreds of visitors requesting and generating dynamic pages from your website over and over again, it will considerably increase the pressure, resulting delayed output and HTTP errors on the client’s browser.
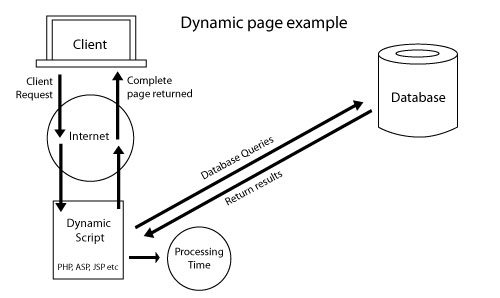
Cached File :— Picture below illustrates how cached files are served instead of dynamic pages, as explained above the cached files are nothing but static web pages. They contain plain HTML code, the only way the content of the cached page will change is if the Web developer manually edits the file. As you can see cached files neither require database connectivity nor the processing time, it is an ideal solution to reduce server pressure and page loading time consistently.
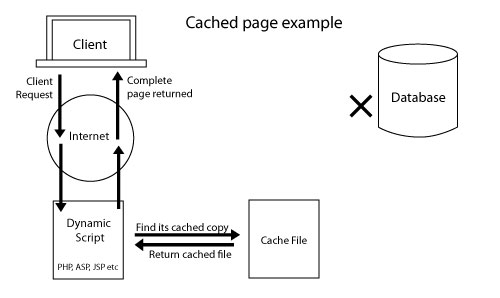
PHP Caching
There are other ways to cache dynamic pages using PHP, but the most common method everyone’s been using is PHP Output Buffer and Filesystem Functions, combining these two methods we can have magnificent caching system.PHP Output buffer :— It interestingly improves performance and decreases the amount of time it takes to download, because the output is not being sent to browser in pieces but the whole HTML page as one variable. The method is insanely simple take a look at the code below :
1
2 3 4 5 6 7 |
<?php
ob_start(); // start the output buffer /* the content */ ob_get_contents(); gets the contents of the output buffer ob_end_flush(); // Send the output and turn off output buffering ?> |
PHP Filesystem :— You may be familiar with PHP file system, it is a part of the PHP core, which allow us to read and write the file system. Have a look at the following code.
1
2 3 |
$fp = fopen('/path/to/file.txt', 'w'); //open file for writing
fwrite($fp, 'I want to write this'); //write fclose($fp); //Close file pointer |
Implementing PHP caching
Now you should be pretty clear about PHP output buffer and filesystem, we can use these both methods to create our PHP caching system. Please have a look at the picture below, the Flowchart gives us the basic idea about our cache system.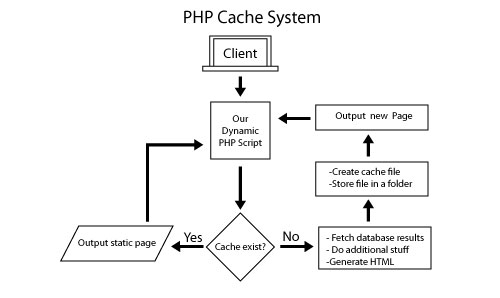
The cycle starts when a user request the content, we just check whether the cache copy exist for the currently requested page, if it doesn’t exist we generate a new page, create cache copy and then output the result. If the cache already exist, we just have to fetch the file and send it to the user browser.
Take a look at the Full PHP cache code below, you can just copy and paste it in your PHP projects, it should work flawlessly as depicted in above Flowchart. You can play with the settings in the code, modify the cache expire time, cache file extension, ignored pages etc.
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
<?php
//settings $cache_ext = '.html'; //file extension $cache_time = 3600; //Cache file expires afere these seconds (1 hour = 3600 sec) $cache_folder = 'cache/'; //folder to store Cache files $ignore_pages = array('', ''); $dynamic_url = 'http://'.$_SERVER['HTTP_HOST'] . $_SERVER['REQUEST_URI'] . $_SERVER['QUERY_STRING']; // requested dynamic page (full url) $cache_file = $cache_folder.md5($dynamic_url).$cache_ext; // construct a cache file $ignore = (in_array($dynamic_url,$ignore_pages))?true:false; //check if url is in ignore list if (!$ignore && file_exists($cache_file) && time() - $cache_time < filemtime($cache_file)) { //check Cache exist and it's not expired. ob_start('ob_gzhandler'); //Turn on output buffering, "ob_gzhandler" for the compressed page with gzip. readfile($cache_file); //read Cache file echo '<!-- cached page - '.date('l jS \of F Y h:i:s A', filemtime($cache_file)).', Page : '.$dynamic_url.' -->'; ob_end_flush(); //Flush and turn off output buffering exit(); //no need to proceed further, exit the flow. } //Turn on output buffering with gzip compression. ob_start('ob_gzhandler'); ######## Your Website Content Starts Below ######### ?> <!DOCTYPE html> <html> <head> <title>Page to Cache</title> </head> <body> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer ut tellus libero. </body> </html> <?php ######## Your Website Content Ends here ######### if (!is_dir($cache_folder)) { //create a new folder if we need to mkdir($cache_folder); } if(!$ignore){ $fp = fopen($cache_file, 'w'); //open file for writing fwrite($fp, ob_get_contents()); //write contents of the output buffer in Cache file fclose($fp); //Close file pointer } ob_end_flush(); //Flush and turn off output buffering ?> |
- Get the currently requested URL location.
- Construct a location path for the cache file, convert URL to MD5 hash for the fixed cache file name.
- Check whether URL is in ignore list.
- Check for existing unexpired cache file, if exist, just open and output the content with gzip compression.
- Or else, we create a new cache file and output the HTML result with gzip compression.
0 comments:
Post a Comment